Java I/O
# Java I/O
# 概述
物质在目的地之间的转移运动称为流,可分为 输入流 、 输出流
输入流: 程序从源中读取数据 输出流: 数据要到达的目的地
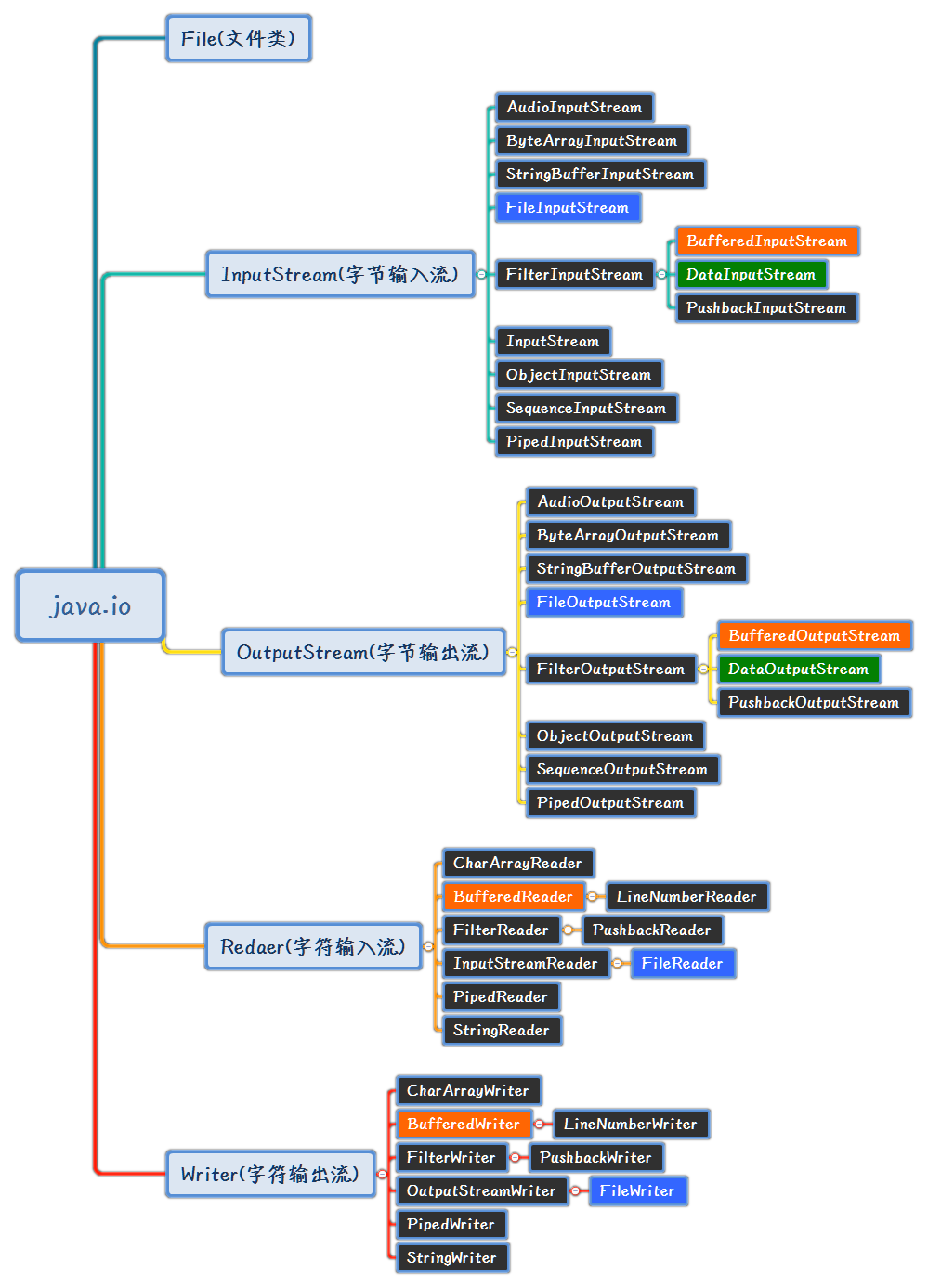
主要流:
文件流 缓冲流 数据流
# 输入流
InputStream类 是字节流 的抽象类,所有字节输入流的父类 java.io.InputStream
Reader类 是字符流 的抽象类 ,是Unicode编码,适合处理文本,所有字符输入流的父类 java.io.Reader
方法 (更多方法自行api)*
返回 | 方法 | 说明 |
---|---|---|
void | close() | 关闭输入流 |
void | mark(int readlimit) | 标记输入流中的当前位置 |
boolean | markSupported() | 支持 mark和 reset方法,则true |
int | read() | 从输入流读取数据,(字节)0~255范围,没有则-1 |
int | read(E[] b) | 从输入流读取一些 字符/字节 ,并将它们存储到缓冲区b |
void | reset() | 将流重新定位到上次输入流调用 mark方法时的位置 |
long | skip(long n) | 跳过输入流上的n个字符,返回实际跳过的字节数 |
# 输出流
OutputStream类 是字节流 的抽象类,所有字节输出流的父类 java.io.OutputStream
Writer类 是字符流 的抽象类 ,所有字符输入流的父类 java.io.Writer
方法 (更多方法自行api)*
返回 | 方法 | 说明 |
---|---|---|
void | close() | 关闭流 |
void | flush() | 完成输出清空缓冲 |
void | write(E text) | 将指定text写入流 |
void | write(E[] b) | 将b数组写入流 |
void | write(E[] b , int off , int len) | 将b数组中从偏移量off开始写入len个字节的流 |
# File类
Class File
java.lang.Object java.io.File
实现接口 Serializable、Comparable
<File>
File类是代表磁盘的 文件 或者 文件夹(目录),该类可实现创建、删除、重命名文件等操作
构造方法
File(String pathname)
抽象路径目录对象(文件夹 或 文件)
File(String parent , String child)
路径目录中的文件(绝对路径)
pathname: 路径名称(包含文件名) parent: 父路径字符串,例如:D://doc 或 D://doc//no1.txt child: 子路径字符串 ,例如:no1.txt
注意:
- 盘符的路径名前缀由驱动器号和
:
组成- 路径分割一般用:
\
或//
- 子路径文件一定要有后缀,如:
.jpg
、.txt
# 文件操作方法
File类提供以下为常用方法 ,如果想看更多方法自行查JDK文档
返回 | 方法 | 说明 |
---|---|---|
String | getName() | 获取文件名称 |
boolean | canRead() | 判断文件是否为可读 |
boolean | canWrite() | 判断文件是否可写入 |
boolean | exists() | 判断文件是否存在 |
long | length() | 获取文件的长度(字节为单位) |
String | getAbsolutePath() | 获取文件的绝对路径 |
String | getParent() | 获取文件的父路径 |
boolean | isFile() | 判断文件是否存在(普通文件) |
boolean | isDirectory() | 判断文件是否为一个目录 |
boolean | isHidden() | 判断文件是否为隐藏文件 |
long | lastModified() | 获取文件最后修改时间(毫秒为单位) |
boolean | createNewFile() | 路径文件不存在,则创建新的空文件 |
boolean | delete() | 删除子路径(最后一个)文件或文件夹 |
boolean | mkdir() | 创建抽象路径名命名的目录(创建文件夹) |
boolean | mkdirs() | 创建抽象路径名命名的目录,包括任何必需但不存在的父目录(创建多层路径文件夹) |
boolean | renameTo(File dest) | 重命名由此抽象路径名表示的文件(移动且替换文件) |
File[] | listFiles() | 返回文件夹所有 子文件夹 和 文件 |
File[] | listFiles(FileFilter filter) | 返回以满足条件的文件夹所有 子文件夹 和 文件 |
# 字节流
FileInputStream、FileOutputStream类 操作磁盘文件的字节
计算机中的所有文件都是二进制形式存储的(文本、图片、···),这些数据可以通过 字节流 与计算机底层的二进制数据进行交互!
# 字节输出流
Class FileOutputStream
java.lang.Object java.io.OutputStream java.io.FileOutputStream
实现接口:
Closeable , Flushable , AutoCloseable
构造方法
FileOutputStream(File file)
FileOutputStream(String fileName)
FileOutputStream(String fileName , boolean append)
FileOutputStream(File file , boolean append)
fileName: 给文件名 file: 抽象路径对象 append: 是否连续写入数据
常用方法 (继承方法点击索引)
返回 | 方法 | 说明 |
---|---|---|
void | write(byte[] b) | 指定字节数组写入文件 |
void | write(byte[] b , int off , int len) | 指定范围写入字节数组 |
void | write(int b) | 将指定字节写入(字节数) |
# 字节输入流
Class FileInputStream
java.lang.Object java.io.InputStream java.io.FileInputStream
实现接口:
Closeable , AutoCloseable
构造方法
FileInputStream(File file)
FileInputStream(String fileName)
fileName: 文件名 file: 要打开阅读的文件
常用方法 (继承方法点击索引)
返回 | 方法 | 说明 |
---|---|---|
long | length() | 返回底层文件的长度 |
int | read() | 从流中读取一字节(读完则 -1 )读完指向下一个字节 |
int | read(byte[] b) | 从流中读取一组字节 |
int | read(byte[] b , int off , int len) | 从流中读取 off 到 len 位置的字节 |
void | seek(long pos) | 将当前流位置为所需位置 |
# 字符流
字符输入输出流可避免像字节流出现乱码现象!他们是使用方法是大致一样!
# 字符输出流
Class FileWriter
java.lang.Object java.io.Writer java.io.OutputStreamWriter java.io.FileWriter
实现接口:
Closeable , AutoCloseable , Flushable , Appendable
构造方法
FileWriter(File file)
FileWriter(String fileName)
FileWriter(String fileName , boolean append)
FileWriter(File file , boolean append)
fileName: 给文件名 file: 抽象路径对象 append: 是否连续写入数据
方法(继承方法点击索引)
传输形式是 以字符为单位进行传输 char
# 字符输入流
Class FileReader
java.lang.Object java.io.Reader java.io.InputStreamReader java.io.FileReader
实现接口:
Closeable , AutoCloseable , Readable
构造方法
FileReader (File file)
FileReader (String fileName)
fileName: 文件名 file: 要打开阅读的文件
方法 (继承方法点击索引)
传输形式是 以字符为单位进行传输 char
# 字节与字符流的转换
# 输出流
Class OutputStreamWriter
java.lang.Object java.io.Writer java.io.OutputStreamWriter
实现接口: Closeable , Flushable , Appendable , AutoCloseable
构造方法
OutputStreamWriter(OutputStream out)
OutputStreamWriter(OutputStream out , Charset cs)
out: 字节输出流 cs: 编码名称
方法 (继承方法点击索引)
返回 | 方法 | 说明 |
---|---|---|
String | getEncoding() | 获取字符编码的名称 |
void | write(char ch) | 写一个字符 |
void | write(char[] ch, int off, int len) | 写数组中的 off 至 len 范围的字符 |
void | write(String str, int off, int len) | 写字符串中的 off 至 len 范围的字符 |
# 输入流
Class InputStreamReader
java.lang.Object java.io.Reader java.io.InputStreamReader
实现接口:
Closeable , AutoCloseable , Readable
构造方法
InputStreamReader(InputStream in)
InputStreamReader(InputStream in, Charset cs)
in: 字节输入流 cs: 编码名称
方法 (继承方法点击索引)
返回 | 方法 | 说明 |
---|---|---|
String | getEncoding() | 获取字符编码的名称 |
int | read() | 读一个字符 |
int | read(char[] ch, int off, int len) | 读数组中的 off 至 len 范围的字符 |
boolean | ready() | 流是否准备好被读取 |
# 缓存字节流
缓存是输入输出流的优化,能大大提升传输效率!
传输形式
文件→字节流→缓存流→目的地
# 缓存字符输出流
Class BufferedOutputStream
java.lang.Object java.io.OutputStream java.io.FilterOutputStream java.io.BufferedOutputStream
构造方法
BufferedOutputStream(OutputStream out)
BufferedOutputStream(OutputStream out , int size)
out: 文件输出流 size: 缓储存大小
以下代码 写入文件
import java.io.*;
public class Demo {
public static void main(String[] args) {
//文件选择大文件为好,容易体现效果
File field = new File("word.txt");
FileOutputStream out = null;
//创建缓存输出流
BufferedOutputStream buffout = null;
//时间记录点1
long a = System.currentTimeMillis();
try {
out = new FileOutputStream(field,true);
//用缓存输出流封装输出流
buffout = new BufferedOutputStream(out);
String str = new String("世界你好!\n");
byte[] bytes = str.getBytes();
//写入99999次
for (int i = 0; i < 99999; i++) {
//单选程序测试
/*
*
* 选择(1)缓存流测试速度
*
* */
//使用流时是使用缓存输出流
buffout.write(bytes);
//刷新。强制将缓冲区数据写入文件中,即使缓冲区没有写满
// 缓存区没写满刷新传输,提升效率
buffout.flush();
/*
*
* 选择(2)字节流测试速度
*
* */
// out.write(bytes);
}
//时间记录点2
long b = System.currentTimeMillis();
System.out.println("运行了:"+(b-a)+"mm");
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
//关闭流
if (out != null){
try {
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (buffout != null){
try {
buffout.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
缓存流输出还是大文件较好,文件选择建议自选大文件为好呈现
# 缓存字符输入流
Class BufferedInputStream
java.lang.Object java.io.InputStream java.io.FilterInputStream java.io.BufferedInputStream
构造方法
BufferedInputStream(InputStream in)
BufferedInputStream(InputStream in , int size)
in:文件输出流 size:缓储存大小
import java.io.*;
public class Demo2 {
public static void main(String[] args) {
//文件选择大文件为好,容易体现效果
File field = new File("word.txt");
FileInputStream input= null;
BufferedInputStream buffin = null;
//时间记录点1
long a = System.currentTimeMillis();
try {
input = new FileInputStream(field);
buffin = new BufferedInputStream(input);
//缓冲区字节数组(与buffered不同)
byte[] bytes = new byte[1024];
/*
*
* 选择(1)缓存流测试速度
*
* */
//直到读完
while ((buffin.read(bytes))!= -1){ }
/*
*
* 选择(2)字节流测试速度
*
* */
//直到读完
// while ((input.read(bytes))!= -1){ }
long b = System.currentTimeMillis();
System.out.println("运行了:"+(b-a)+"mm");
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (input != null){
try {
input.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (buffin != null){
try {
buffin.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
运行结果 当然是缓存流比字节流快啦,前提是大文件!
# 缓存字符流
可以以行为单位进行 输入/输出 ,与以上的缓冲字符流大部分相同!
# 缓存字符输出流
Class BufferedWriter
java.lang.Object java.io.Writer java.io.BufferedWriter
构造方法与以上的一样!
缓存字符输出流提供独有方法:newLine()
写入一行(换行) 带分割符
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
public class Demo {
public static void main(String[] args) {
File file = new File("word.txt");
FileWriter fw= null;
BufferedWriter bfw = null;
try {
//先创建后关闭
fw = new FileWriter(file);
bfw = new BufferedWriter(fw);
String str = new String("世界这么大,");
String str2 = new String("我想去看看。");
bfw.write(str);//写入数据
bfw.newLine();//创建新行
bfw.write(str2);//写入数据
} catch (IOException e) {
e.printStackTrace();
} finally {
//先创建后关闭
if (bfw != null){
try {
bfw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fw != null){
try {
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
在项目创建了test.txt文件,并写入数据 "世界这么大,\n我想去看看。"
# 缓存字符输入流
Class BufferedReader
java.lang.Object java.io.Reader java.io.BufferedReader
缓存字符输入流提供独有方法:String readLine()
读取一个文本行
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class Demo2 {
public static void main(String[] args) {
File file = new File("word.txt");
FileReader fr = null;
BufferedReader bfr = null;
try {
fr = new FileReader(file);
bfr = new BufferedReader(fr);
String tmp = null;
//计数变量
int i = 1 ;
//逐行获取字符串并输出
while ((tmp = bfr.readLine()) != null){
System.out.println("第"+(i++)+"行:"+tmp);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (bfr != null){
try {
bfr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (fr != null){
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
/*
第1行:世界这么大,
第2行:我想去看看。
*/
# 数据流
允许应用程序以与机器无关的方式从底层输入流中读取基本java数据类型
# 数据输出流
Class DataOutputStream
java.lang.Object java.io.OutputStream java.io.FilterOutputStream java.io.DataOutputStream
构造方法
DataOutputStream(OutputStream out)
out:底层输出流,保存供以后使用
DataOutputStream类提供了以下独有方法
修饰符 | 方法 | 说明 |
---|---|---|
void | writeBoolean(boolean v) | 将 boolean写入底层输出流(1字节) |
void | writeByte(int v) | 将 byte值写入底层输出流(1字节) |
void | writeBytes(String s) | 将字符串作为字节序列写入基础输出流 |
··· | ··· | ···多余自行查询 JDK文档 |
void | writeUTF(String str) | 使用 modified UTF-8编码以机器无关的方式将字符串写入基础输出流 |
# 数据输入流
Class DataInputStream
java.lang.Object java.io.InputStream java.io.FilterInputStream java.io.DataInputStream
构造方法
DataInputStream(InputStream in)
in:指定的输入流
import java.io.*;
public class Demo {
public static void main(String[] args) {
File file = new File("word.txt");
//创建字节流
FileOutputStream out = null;
FileInputStream inp = null;
//创建数据流
DataOutputStream dataout = null;
DataInputStream datainp = null;
try {
out = new FileOutputStream(file);
dataout = new DataOutputStream(out);
inp = new FileInputStream(file);
datainp = new DataInputStream(inp);
//写入数据
dataout.writeUTF("使用writeUTF方法写入!");
dataout.writeChars("使用writeChars方法写入!");
dataout.writeBytes("使用writeBytes方法写入!");
//读取数据
System.out.println("在输入流读取数据:"+datainp.readUTF());
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (datainp != null){
try {
datainp.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (inp != null){
try {
inp.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (dataout != null){
try {
dataout.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (out != null){
try {
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
/* 控制台结果
在输入流读取数据:使用writeUTF方法写入!
*/
# 打印流
Class PrintWriter
java.lang.Object java.io.Writer java.io.PrintWriter
实现接口:
Closeable , Flushable , Appendable , AutoCloseable
将对象的格式表示打印到文本输出流,打印过程不需刷新!
构造方法 (更多方法自行API)
PrintWriter(File file)
PrintWriter(OutputStream out)
PrintWriter(String fileName)
PrintWriter(Writer out)
方法 (继承方法点击索引)
返回 | 方法 | 说明 |
---|---|---|
PrintWriter | append(char ch) | 指定字符追加 |
boolean | checkError() | 检查流是否关闭 |
void | clearError() | 清除流中的错误状态 |
void | print(E) | 写入数据(支持多类型,详细API) |
void | println(E) | 写入数据末尾换行(支持多类型,详细API) |
void | write(···) | 继承输入流写入的方法 |
# NIO
NIO支持面向缓冲区的、基于通道的IO操作。NIO将以更加高效的方式进行文件的读写操作
Java NIO 由以下几个核心部分组成:
- 通道 Channels
- 缓存区 Buffers
# 缓存区 Buffers
缓存区 Buffer 主要用于与 NIO 通道进行交互,数据是从通道读入缓冲区,从缓冲区写入通道中的
缓存区 本质是个 读写 的内存,而内存被封装为NIO Buffer对象
Buffer实现类: ByteBuffer、MappedByteBuffer、CharBuffer、DoubleBuffer、FloatBuffer、IntBuffer、LongBuffer、ShortBuffer
Buffer对象属性
- **capacity 容量:**Buffer 的内存块(容量),缓冲区容量不能为负,并且创建后不能更改
- limit 限制: 剩余可写容量的指针
- 在 写模式 下,最多能写入 limit个单元 的数据
- 在 读模式 下,最多能够读取 limit个单元 的数据,一旦切换为读,就要读完,否则会被舍弃!
- 当模式从 写 切换至 读 时,limit 被设置成写模式下的position值,而position重置为0
- limit = capacity – (position + 1)
- position 位置: 位置操作的指针
- 当 写入数据 时,position会移到下入的下一个Buffer单元
- 当 读取数据 时,Buffer切换至模式,position会重置为0,读数据时position会下移读取可读数据
- position位置指标 最大值为 capacity - 1
Buffer常用方法
allocate()
开辟新缓存空间clear()
清缓存。limit和position为零array()
获取缓存区数据flip()
使缓冲区准备好新的通道写入 获取操作 序列。limit为position,position为零rewind()
使缓冲区准备好重新读取已经包含的数据。limit保持不变,position为零
Buffers应用步骤:
- 分配缓存区内存
allocate(int length)
静态方法 - 写入数据,Buffer对象 调用
put()
方法 写入内存 - 切换模式,切换至 读/写 ,Buffer对象 调用
flip()
方法(position重置为0 - 读取数据,Buffer 中读取数据
get()
、通过数据批获取 - 清缓存,Buffer对象 调用
clear()
方法
示例:
public static void testBuffer() {
//创建分配容量长度为10的字节缓冲区
ByteBuffer buf = ByteBuffer.allocate(10);
System.out.println("position="+buf.position()); //0
System.out.println("limit="+buf.limit()); //10
System.out.println("capacity="+buf.capacity()); //10
String putMsg="abcd";
//将字符串转换成字节数组
System.out.println("将abcd字符串存储到bytebuffer");
buf.put(putMsg.getBytes(), 0, putMsg.getBytes().length);
System.out.println("position="+buf.position()); //4
System.out.println("limit="+buf.limit()); //10
System.out.println("capacity="+buf.capacity()); //10
//翻转成读模式
System.out.println("翻转成读模式----------");
buf.flip();
System.out.println("position="+buf.position()); //0
System.out.println("limit="+buf.limit()); //4
System.out.println("capacity="+buf.capacity()); //10
byte[] cbuf = new byte[buf.limit()];
//从缓存区读取数据
System.out.println("从缓存区读取数据");
buf.get(cbuf, 0, cbuf.length);
System.out.println(new String(cbuf,0,cbuf.length));
// abcd
buf.clear();
}
# 通道 Channels
通道 Channels 是针对目标文件打开的连接,而建立的一个通道
- 双向通道。读写数据都是通过通道进行数据交互。但流的读写通常是单向的
- 通道可以异步地读写
- 通道中的数据总是要先读到一个Buffer,或者总是要从一个Buffer中写入
通道实现类:
- 文件读写 FileChannel
- UDP网络读写 DatagramChannel
- TCP网络读写 SocketChannel
- 监听连接的TCP ServerSocketChannel
# 文件读写 FileChannel
FileChannel 应用步骤:
- 获取文件指定的 字节输入流
- 流转换 FileChannel对象,字节输入流 调用
getChannel()
方法 - 创建缓存区(类型根据情况选择
- 循环读取直至为空
- 每次循环都要缓存都要
flip()
反转 (以便控制读取范围 - 每次循环读取后都要清空内存
- 每次循环都要缓存都要
示例:
public static void testFileChannel() throws IOException {
//创建FileInputStream对象
FileInputStream fileInputStream=new FileInputStream(new File("a.txt"));
//获取FileChannel对象
FileChannel fc = fileInputStream.getChannel();
ByteBuffer buf=ByteBuffer.allocate(12);
while(fc.read(buf) != -1) {
//设置ButeBuffer为读取模式
buf.flip();
String message=new String(buf.array(),0,buf.limit());
System.out.print(message);
buf.clear();
}
fileInputStream.close();
}
读写拷贝应用实例:
public static void testCopyFile01() throws IOException {
long start = System.currentTimeMillis();
FileInputStream srcFileInputStream=new FileInputStream(new File("E:\\资源\\巨人三\\1.mp4"));
FileOutputStream destFileOutputStream=new FileOutputStream(new File("E:\\资源\\巨人三\\test\\2.mp4"));
//获取针对1.mp4文件的FileChannel对象
FileChannel fc=srcFileInputStream.getChannel();
FileChannel destFc=destFileOutputStream.getChannel();
//创建ByteBuffer对象
//使用非直接方式创建ByteBuffer
ByteBuffer buf = ByteBuffer.allocate(1024);
while(fc.read(buf)!=-1) {
//配置ByteBuffer的可读模式
buf.flip();
destFc.write(buf);
//清除缓存区
buf.clear();
}
long end = System.currentTimeMillis();
System.out.println("耗费时间为:" + (end - start));
fc.close();
destFc.close();
srcFileInputStream.close();
destFileOutputStream.close();
}
public static void testCopyFile02() throws IOException {
long start = System.currentTimeMillis();
//建立1.mp4的通道(就是连接点)
FileChannel fileChannelA=FileChannel.open(Paths.get("E:\\资源\\巨人三\\1.mp4"), StandardOpenOption.READ);
FileChannel fileChannelB=FileChannel.open(Paths.get("E:\\资源\\巨人三\\test\\2.mp4"),
StandardOpenOption.WRITE,
StandardOpenOption.READ,
StandardOpenOption.CREATE);
//建立fileChannelA通道的映射内存
MappedByteBuffer inMappedBuf = fileChannelA.map(FileChannel.MapMode.READ_ONLY, 0, fileChannelA.size());
//建立fileChannelB通道的映射内存
MappedByteBuffer outMappedBuf = fileChannelB.map(FileChannel.MapMode.READ_WRITE, 0, fileChannelA.size());
//创建一个字节数组
byte[] dst = new byte[inMappedBuf.limit()];
inMappedBuf.get(dst);
outMappedBuf.put(dst);
long end = System.currentTimeMillis();
System.out.println("耗费时间为:" + (end - start));
//关闭通道
fileChannelA.close();
fileChannelB.close();
}
# 代码实例
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Demo {
public static void main(String[] args) throws IOException {
File f = new File("src/","doc.txt");
//会在项目里的根路经的src文件夹里常见doc.txt文件
System.out.println("文件不存在,则创建新文件? "+f.createNewFile());
System.out.println("获取文件名称:"+f.getName());
System.out.println("文件是否可读? "+f.canRead());
System.out.println("文件是否可写? "+f.canWrite());
System.out.println("判断文件是否存在? "+f.exists());
System.out.println("输出文件长度:"+f.length());
System.out.println("输出文件路径:"+f.getAbsolutePath());
System.out.println("输出文件父路径:"+f.getParent());
System.out.println("判断文件是否存在(普通文件)?"+f.isFile());
System.out.println("文件是否为一个目录?"+f.isDirectory());
System.out.println("文件是否为隐藏文件?"+f.isHidden());
System.out.println("输出文件最后修改时间戳:"+f.lastModified());
Date date = new Date(f.lastModified());
SimpleDateFormat sdf = new SimpleDateFormat("yy/MM/dd HH:mm");
System.out.println("输出文件最后修改时间:"+sdf.format(date));
// System.out.println("删除文件?"+f.delete());
//创建的是文件夹
// mkdir (创建单个)
// mkdirs (创建多个)
System.out.println("创建抽象路径名命名的目录?"+f.mkdir());
//创建在与项目根路经
File fm = new File("cs1/cs2/cs3/");
System.out.println("创建抽象路径名命名的目录?"+fm.mkdirs());
System.out.println("===================");
//listFiles方法测试
//项目根路经
File file = new File("");
//获取项目根路经(防止空指针异常)
File file2 = new File(file.getAbsolutePath());
//file2抽象路径必须为绝对路径否则空指针异常
File[] files = file2.listFiles();
for (File tmp : files){
if (tmp.isFile()){
System.out.println("文件:"+tmp.getName());
}else{
System.out.println("文件夹:"+tmp.getName());
}
}
}
}
/*
文件不存在,则创建新文件? false
获取文件名称:doc.txt
文件是否可读? true
文件是否可写? true
判断文件是否存在? true
输出文件长度:0
输出文件路径:E:\Java\Study\15Chapter (输入输出流)\15.3.2 File方法\src\doc.txt
输出文件父路径:src
判断文件是否存在(普通文件)?true
文件是否为一个目录?false
文件是否为隐藏文件?false
输出文件最后修改时间戳:1594525019225
输出文件最后修改时间:20/07/12 11:36
创建抽象路径名命名的目录?false
创建抽象路径名命名的目录?false
===================
文件夹:.idea
文件:15.3.2 File方法.iml
文件夹:cs1
文件夹:out
文件夹:src
*/
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
public class Demo {
public static void main(String[] args) {
//FileOutputStream输出流数据(写入数据)
//项目根路经
File f = new File("");
//获取项目根路经(防止空指针异常)
File file = new File(f.getAbsolutePath(),"test.txt");
FileOutputStream out = null;
try {
out = new FileOutputStream(file,true);
//判断文件是否存在
if (!file.exists()){
//新建文件
file.createNewFile();
}
//将字符串转换为字节数组
String str = "我喜欢学习Java编程语言!";
byte[] bytes = str.getBytes();
//写入数据
out.write(bytes);
} catch (Exception e){
e.printStackTrace();
}finally {
try {
//关闭流
if (out != null){
out.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
/*
在项目根目录 src 里的 Test.txt文件 写入内容
*/
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public class Demo2 {
public static void main(String[] args) {
//FileInputStream字节输入流(读取文件)
//项目根路经
//获取项目根路经(防止空指针异常)
File file = new File(new File("").getAbsolutePath(),"test.txt");
FileInputStream input = null;
try {
input = new FileInputStream(file);
//判断文件是否存在
if (!file.exists()){
//新建文件
file.createNewFile();
}
//每次读取1KB (1字节 = 1B = 1024 KB )
byte[] bytes = new byte[1024];
//第一次读取
int len = input.read(bytes);
//存储读取的数据
StringBuilder src = new StringBuilder();
while (len != -1){
src.append(new String(bytes , 0 , len));
len = input.read(bytes);
}
System.out.println("文件内容为:"+src);
}catch (Exception e){
e.printStackTrace();
}finally {
try {
//关闭流
if (input != null){
input.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
/*
在项目根目录 src 里的 Test.txt文件 读取内容
*/
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
public class Demo2 {
public static void main(String[] args) {
//输出(写入)
File file = new File("word.txt");
FileWriter fw = null;
try {
fw = new FileWriter(file);
String str = new String("欢迎来到,我的世界");
fw.write(str);
fw.append(",").append("Java!");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fw != null) {
fw.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
/*
在项目根目录 src 里的 Test.txt文件 写入内容
*/
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class Demo {
public static void main(String[] args) {
//输入 (读取)
File file = new File("word.txt");
FileReader fr = null;
try {
fr = new FileReader(file);
char[] ch = new char[1024];
int len = fr.read(ch);
StringBuilder src = new StringBuilder();
while (len != -1) {
//循环读取
src.append(new String(ch, 0, len));
len = fr.read(ch);
}
System.out.println("文件内容: " + src);
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fr != null) {
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
/*
在项目根目录 src 里的 Test.txt文件 读取内容
*/